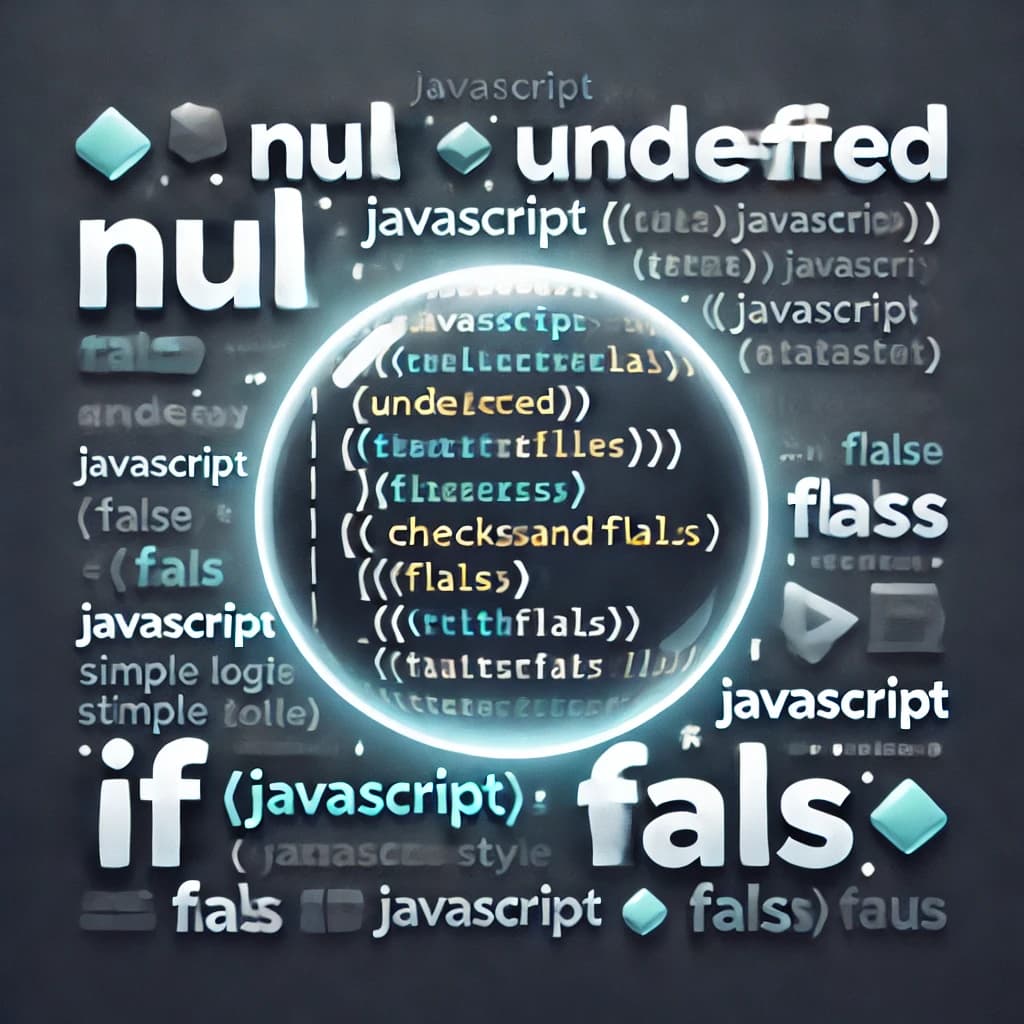
Truthy and Falsy Values in JavaScript
Mahmudul Hasan Nayeem / September 24, 2024
In JavaScript, every value is inherently either "truthy" or "falsy." Understanding the difference between these two concepts is essential for writing efficient code, especially when dealing with conditions like if statements, loops, or other logical operations. In this blog, we will dive into what truthy and falsy values are, explore examples, and discuss why these concepts matter in JavaScript.
What are Truthy and Falsy Values?
In JavaScript, truthy and falsy values determine how a value is evaluated in a
boolean context, such as conditions in if
statements. Here's a quick
breakdown:
-
Truthy Values: Any value that is not falsy is considered truthy. This includes values like
true
,1
,"hello"
,[]
,{}
, and even functions. When evaluated in a boolean context, truthy values returntrue
. -
Falsy Values: Values that are considered falsy include
false
,0
,""
,null
,undefined
, andNaN
. When evaluated in a boolean context, falsy values returnfalse
.
These values play a significant role in conditional logic, and JavaScript
implicitly converts them to true
or false
depending on their type.
Falsy Values in JavaScript
A falsy value is a value that, when converted to a boolean, evaluates to
false
. There are only a handful of falsy values in JavaScript:
false
: The boolean valuefalse
is always falsy.0
: The number0
is considered falsy.""
: An empty string is falsy (""
or''
or `` (empty string) – Any string with no characters.).null
: Thenull
value is falsy.undefined
: Theundefined
value is falsy.NaN
: TheNaN
value (Not-a-Number) is falsy.-0
: Negative zero is also falsy (rarely used, but still falsy).
Example
if (!0) {
console.log('0 is falsy') // This will execute
}
if (!'') {
console.log('Empty string is falsy') // This will execute
}
In this example, both 0
and an empty string (""
) evaluate to falsy, so the
if
statements are triggered.
Truthy Values in JavaScript
Any value that is not falsy is considered truthy. This includes:
- The boolean value
true
is always truthy. - Any non-zero number (positive or negative)
- Arrays and objects (including empty ones)
- Any non-zero number (positive or negative)
Example
if (42) {
console.log('42 is truthy') // This will execute
}
if ('Hello World') {
console.log('Non-empty string is truthy') // This will execute
}
if ([]) {
console.log('Empty array is truthy') // This will execute
}
In this example, the values 42
, "Hello World"
, and []
(empty array) are
truthy, so the conditions in the if
statements evaluate to true
.
Common Use Cases for Truthy and Falsy Values
1. Default Parameter Values:
In functions, you can assign default values using truthy and falsy checks.
function greet(name) {
name = name || 'Guest'
console.log(`Hello, ${name}!`)
}
greet() // Outputs: Hello, Guest!
greet('Mahmudul') // Outputs: Hello, Mahmudul!
If no name
is provided, the falsy value undefined
is passed, so
name || 'Guest'
evaluates to 'Guest'
.
2. Checking for Empty Objects or Arrays:
Although an empty object or array is truthy, its length or properties can be checked for content:
let arr = []
if (arr.length) {
console.log('Array is not empty')
} else {
console.log('Array is empty') // This will execute
}
3. Conditional Rendering:
In many front-end frameworks, like React, truthy and falsy values are often used to conditionally render elements.
let isLoggedIn = true
return <div> {isLoggedIn && <p>Welcome back, user!</p>} </div>
In this example, if isLoggedIn
is truthy, the welcome message is displayed.
Key Takeaways:
- Falsy values:
false
,0
,""
,null
,undefined
,NaN
,-0
. - Truthy values: Any value that is not falsy.
0